File Actions
The File Actions Library provides a robust set of actions for interacting with files throughout your automated tests. These actions are useful for handling test data stored in files or for interacting with files during test automation.
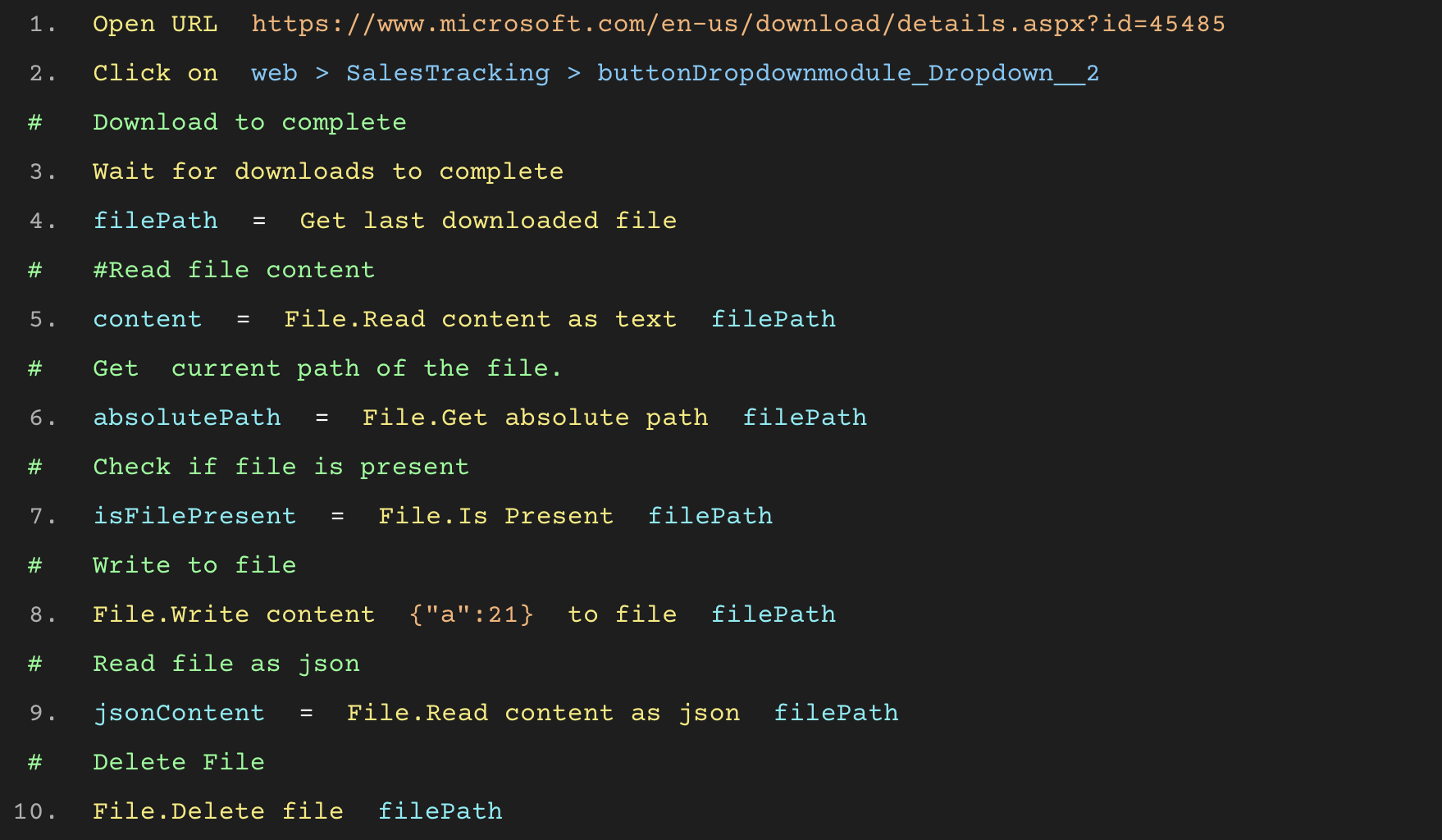
Action | Description |
---|---|
File.Read content as text (filePath) | Reads the content of a specified file and returns it as plain text. |
File.Read content as JSON (filePath) | Reads the content of a specified file, parses it as JSON data. |
File.Write (content) to (filePath) | Writes the provided content to a specified file. |
File.Is Present (filePath) | Checks if a file exists at the specified path. |
File.Delete file (filePath) | Deletes the specified file. |
File.Get absolute path (filePath) | Retrieves the absolute path of a file. |
File.Read content as text (filePath)
This action reads the content of the file located at the given file path and returns it as plain text.
-
Usage:
content = File.Read content as text("/path/to/file.txt")
-
Arguments:
- filePath: The path to the file that needs to be read.
-
Returns:
- Returns the file's content as a text string.
File.Read content as JSON (filePath)
This action reads the content of the file located at the given file path and returns it as JSON.
-
Usage:
jsonContent = File.Read content as JSON("/path/to/file.txt")
-
Arguments:
- filePath: The path to the file that needs to be read.
-
Returns:
- Returns the file's content as a Json.
File.Write (content) to (filePath)
This action writes the provided content to the specified file. If the file does not exist, it will be created.
-
Usage:
File.Write content {"a":21} to file filePath
-
Arguments:
- content: The data to be written to the file.
- filePath: The path of the file where the content should be written.
File.Is Present (filePath)
This action checks if a file exists at the specified path.
-
Usage:
isFilePresent = File.Is Present("/path/to/file.txt");
-
Arguments:
- filePath: The path of the file where the content should be written.
File.Delete file (filePath)
This action deletes the file located at the specified path.
-
Usage:
File.Delete file("/path/to/file.txt");
-
Arguments:
- filePath: The path of the file where the content should be written.
File.Get absolute path (filePath)
This action retrieves the absolute path of a file, converting any relative path to an absolute path based on the current location.
-
Usage:
absolutePath = File.Get absolute path "./config/settings.json"
In this example, the relative path to settings.json is converted to its absolute path and stored in the absolutePath variable.
-
Arguments:
- filePath: The path of the file where the content should be written.